Looking to add powerful image description capabilities to your application? Easy-Peasy.AI’s Image Description API transforms any image into detailed, accurate descriptions using advanced AI. This guide shows you how to integrate this feature into your projects.
Getting Started
1. Get Your API Key
- Sign up for an Easy-Peasy.AI account
- Visit your API settings page
- Copy your unique API key
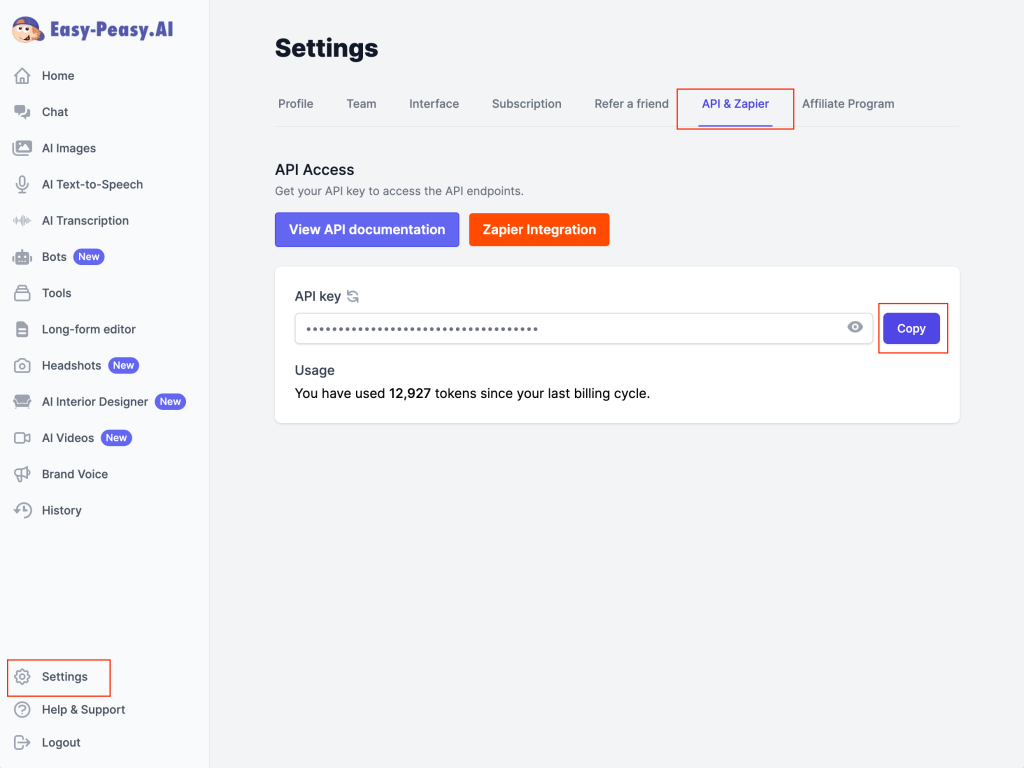
2. API Endpoint
POST https://easy-peasy.ai/api/generate
Code Examples
Python Implementation
import requests
import json
def get_image_description(api_key, image_url, extra_instructions=None):
url = "https://easy-peasy.ai/api/generate"
headers = {
"accept": "application/json",
"x-api-key": api_key,
"Content-Type": "application/json"
}
payload = {
"preset": "image-description-generator",
"keywords": image_url
}
if extra_instructions:
payload["extra1"] = extra_instructions
response = requests.post(url, headers=headers, json=payload)
if response.status_code == 200:
return response.json()
else:
raise Exception(f"Error: {response.status_code}, {response.text}")
# Example usage
api_key = "YOUR_API_KEY"
image_url = "https://example.com/image.jpg"
result = get_image_description(api_key, image_url)
print(result)
JavaScript/Node.js Implementation
// Using fetch
async function getImageDescription(apiKey, imageUrl, extraInstructions = null) {
const url = 'https://easy-peasy.ai/api/generate';
const payload = {
preset: 'image-description-generator',
keywords: imageUrl
};
if (extraInstructions) {
payload.extra1 = extraInstructions;
}
try {
const response = await fetch(url, {
method: 'POST',
headers: {
'accept': 'application/json',
'x-api-key': apiKey,
'Content-Type': 'application/json'
},
body: JSON.stringify(payload)
});
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
return await response.json();
} catch (error) {
console.error('Error:', error);
throw error;
}
}
// Example usage
const apiKey = 'YOUR_API_KEY';
const imageUrl = 'https://example.com/image.jpg';
getImageDescription(apiKey, imageUrl)
.then(result => console.log(result))
.catch(error => console.error('Error:', error));
PHP Implementation
<?php
function getImageDescription($apiKey, $imageUrl, $extraInstructions = null) {
$url = 'https://easy-peasy.ai/api/generate';
$payload = [
'preset' => 'image-description-generator',
'keywords' => $imageUrl
];
if ($extraInstructions) {
$payload['extra1'] = $extraInstructions;
}
$ch = curl_init($url);
curl_setopt_array($ch, [
CURLOPT_RETURNTRANSFER => true,
CURLOPT_POST => true,
CURLOPT_HTTPHEADER => [
'accept: application/json',
'x-api-key: ' . $apiKey,
'Content-Type: application/json'
],
CURLOPT_POSTFIELDS => json_encode($payload)
]);
$response = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
if (curl_errno($ch)) {
throw new Exception(curl_error($ch));
}
curl_close($ch);
if ($httpCode !== 200) {
throw new Exception("HTTP Error: $httpCode, Response: $response");
}
return json_decode($response, true);
}
// Example usage
try {
$apiKey = 'YOUR_API_KEY';
$imageUrl = 'https://example.com/image.jpg';
$result = getImageDescription($apiKey, $imageUrl);
print_r($result);
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
Customization Options
Product Descriptions
{
"preset": "image-description-generator",
"keywords": "YOUR_IMAGE_URL",
"extra1": "Write the product description based on the uploaded image"
}
Detailed Analysis
{
"preset": "image-description-generator",
"keywords": "YOUR_IMAGE_URL",
"extra1": "Provide a detailed analysis of the image including colors, composition, and mood"
}
Best Practices
- Error Handling: Always implement proper error handling
- Rate Limiting: Monitor your API usage
- Image URLs: Use publicly accessible image URLs
- Security: Never expose your API key in client-side code
- Validation: Validate image URLs before sending to the API
Response Handling
Status codes:
- 200: Success
- 400: Bad Request
- 401: Unauthorized
- 429: Too Many Requests
- 500: Server Error
Use Cases
E-commerce
- Product Catalogs: Automatically generate SEO-friendly product descriptions from product images
- Alt Text Generation: Create accessible alt text for product images
- Inventory Management: Extract product details from images for database entries
Content Management
- Media Libraries: Auto-tag and describe images in content management systems
- Blog Platforms: Generate image captions and descriptions for blog posts
- Social Media: Create engaging descriptions for visual content
Accessibility
- Web Accessibility: Generate WCAG-compliant image descriptions
- Screen Reader Support: Provide detailed descriptions for visually impaired users
- Educational Materials: Create descriptive text for educational resources
Digital Marketing
- SEO Optimization: Generate keyword-rich image descriptions
- Social Media Marketing: Create engaging captions for visual content
- Content Automation: Streamline content creation workflows
AI Applications
- Visual Search: Enable detailed image-based search functionality
- Content Moderation: Analyze and describe image content for moderation
- Data Analysis: Extract insights from visual content
Need Help?
- Email: support@easy-peasy.ai
- Documentation: API Documentation
Start generating accurate image descriptions today with Easy-Peasy.AI’s powerful API!